Introduction
Premise and basic concepts
The following documentation is intended to illustrate the functionalities related to the API REST exposed by the system.
In order to easily use the API REST, it is necessary to have some basic notions about core concepts and objects.
If you already have these competencies, you can skip this chapter and proceed further.
Web API Controllers
The API REST communication functionalities are implemented through special Web API Controllers.
Each Controller contains a set of features called Actions.
Each Action constitutes an end-point of the system, and can be invoked via HTTP via a URL unique and a specific set of HTTP verbs
The section on Routing illustrates the ways in which it is possible to invoke the Actions of Controller
Business Objects
The objects that represent the entities of the system (companies, contacts, leads, opportunities, activities, etc.) are called "Business Objects" (indicated for brevity with the acronym "BO")
The complete list of Business Objects is formalized in the appropriate enum "BusinessObjectType"
Each element of the enum BusinessObjectType matches a specific Business Object
Business Objects Controllers
Business Objects are the heart of the system's business logic, and expose their functions through special API REST end-points called "Web API controllers "
In order to interact with a Business Object, it is therefore necessary to identify the corresponding end-point. The Controllers section contains the list of all available controllers, indicating the type for each one.
The Controllers related to a specific Business Object are marked with the icon
The section on Routing illustrates the ways in which it is possible to invoke the Actions of the Controller
DTO (Data Transfer Objects)
Business Objects are very complex entities, rich in information and functions.
It would be impractical to directly manipulate them via the Actions of Controllers (for the obvious reason that objects and services are programmatic entities with very different usage semantics)
To facilitate their usage (and in particular, to simplify their JSON representation), for each Business Object there is a correspondent DTO, a "Data Transfer Object".
The purpose of a DTO is to represent, serialize and transport the data of a Business Object.
The Actions of the Controllers receive (parameters) and returns JSON data of the DTO objects
As previously indicated, the complete list of Business Objects is formalized in the appropriate enum "BusinessObjectType"
Each element of the enum BusinessObjectType matches the DTO of a specific Business Object
The JSON⇄DTO⇄BO "transformation chain"
As previously said, the BOs are manipulated via JSON through the "mediation" of the DTOs.
The following diagrams illustrate (in a very simplified way) the pipeline "connecting" the invoking client (on the left) to the database of the backend (on the right) through the JSON⇄DTO⇄BO "transformation chain"
Serialization (JSON←DTO←BO)
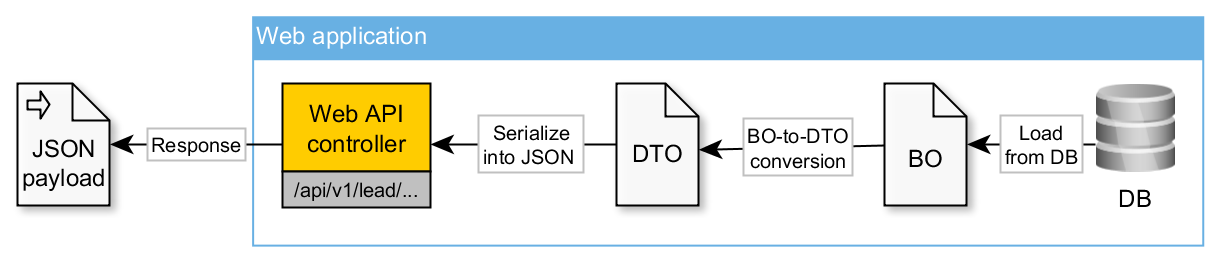
Deserialization (JSON→DTO→BO)
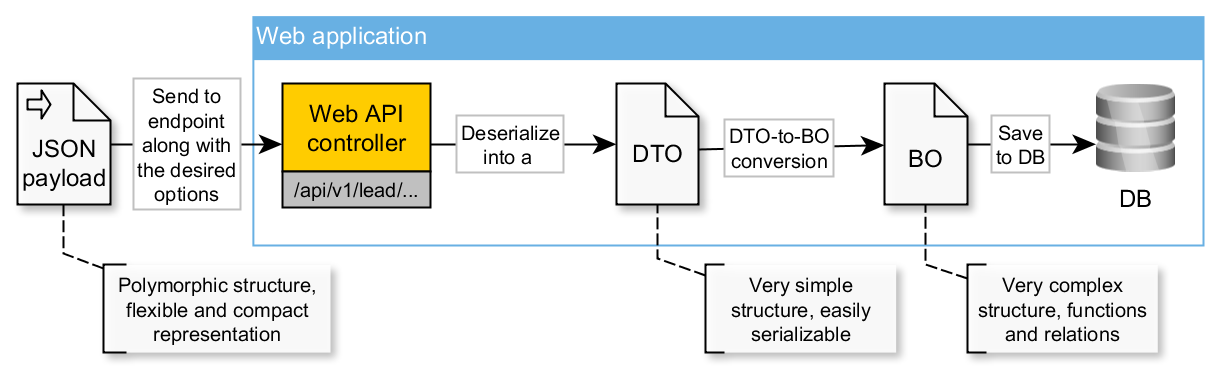
Authentication
JWT Token
In order to invoke the REST API, it is necessary to obtain an authentication token via the appropriate service /Auth/Login, to which a JSON package must be sent in the following form:
{
"grant_type": "password",
"username": "admin@admin.com",
"password": "admin"
}
The service will return a JWT token (access_token property) to be used in all subsequent calls using Bearer Authentication
{
"access_token": "my_jwt_token",
"expires_in": 1200,
"refresh_token": "5fc87399-413d-44de-8976-dc3d15e4b0bc",
"result": 0,
"token_type": "bearer"
}
The communication flow is as follows:
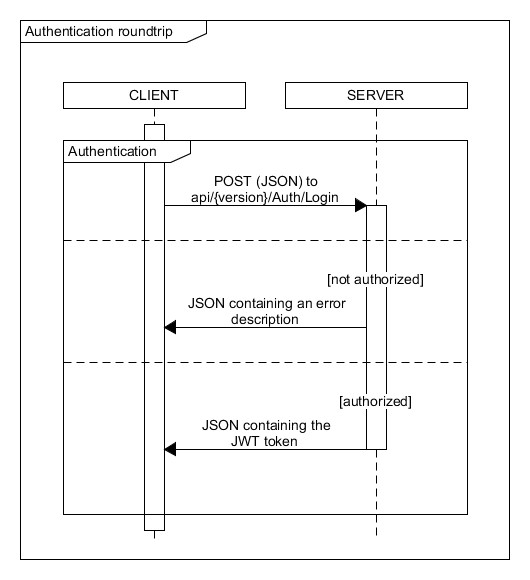
Bearer Authentication
Bearer authentication (also called token authentication) is an HTTP authentication scheme that involves security tokens called bearer tokens.
The name “Bearer authentication” can be understood as “give access to the bearer of this token.”
The bearer token is a cryptic string or hash, usually generated by the server in response to a login request.
The client must send this token in the Authorization header when making requests to protected endpoints/resources:
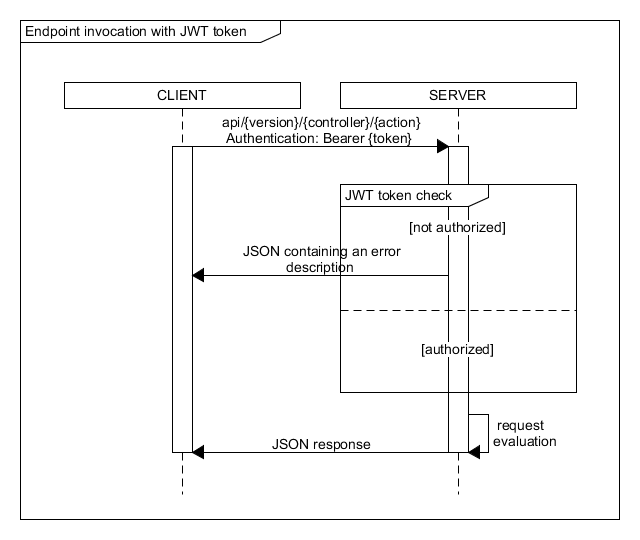
The Bearer authentication scheme was originally created as part of OAuth 2.0 in RFC 6750, but is sometimes also used on its own. Similarly to Basic authentication, Bearer authentication should only be used over HTTPS (SSL).
A typical HTTP GET invocation of a protected REST API endpoint/resource is similiar to this:
GET https://myhost.com/api/latest/Company/Get/1 HTTP/1.1
Host: myhost.com
Connection: keep-alive
Accept: application/json, text/plain, */*
Origin: null
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64)
Authorization: Bearer my_jwt_token
Accept-Encoding: gzip, deflate, br
Accept-Language: en,en-US;q=0.9,it-IT;q=0.8,it;q=0.7
API Key
API keys are a simple encrypted string that can be used when calling certain API for authentication.
To generate an API key token, go to SETUP => USERS => USER.
Open the TAB "Web API Keys" and click the button Add Web API Key to generate a new token.
Each token can have a specific security to limit the actions on the main object.
For example, to allow new leads creation only, we recommend disabling all features except the flag "create" on the lead line.
Pass the API key into a REST API call as a header or query parameter with the following format. Replace API_KEY with your API key,
apikey=API_KEY
For example, to pass an API key for a Lead's new Instance request:
GET http://api.crmincloud.it/api/latest/lead/GetNewInstance?apikey=API_KEY
Identification of the calling application
Some of the REST API functions can only be used if (in addition to proper user authentication) a declaration of the calling application is also performed.
The name of the calling application is sent to the server via a special HTTP header:
Crm-ApplicationName
If you have this "Application Name", use it in all calls to the server.
GET https://myhost.com/api/latest/Company/Get/1 HTTP/1.1
Host: myhost.com
Connection: keep-alive
Accept: application/json, text/plain, */*
Origin: null
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64)
Authorization: Bearer my_jwt_token
Accept-Encoding: gzip, deflate, br
Accept-Language: en,en-US;q=0.9,it-IT;q=0.8,it;q=0.7
Crm-ApplicationName: MyApplication
Controllers
The following list contains all the available Web API controllers
- Account
- Activity
- Activity Sub Type
- Address
- Anagraphic Company Type
- Anagraphic Industry
- Anagraphic Interest
- Anagraphic Priority
- Anagraphic Rating
- Anagraphic Source
- Anagraphic Status
- Appointment
- Auth
- Calendar Configuration
- Calendar Event
- Catalog
- Catalog Categories
- Catalog Product Price
- Company
- Contact
- Contact Category
- Currency
- EMail Type
- Flow
- Flow Template
- Flow Trigger
- Groups
- Hook
- Lead
- List
- Mail Category
- Mailing
- Mailing Delivery
- Mailing Domain
- Mail Template
- Message
- Message Channel
- Opportunity
- Opportunity Category
- Opportunity Lost Reason
- Opportunity Phase
- Order
- Payment
- Payment Mode
- Phone Type
- Price List Description
- Privacy Activity Motivation Implicit Consent
- Privacy Management
- Privacy Motivations Implicit Consent
- Privacy Type Management
- Progressive Code
- Quote
- Referrer Category
- Relation Type
- Sales Performance Target
- Signature
- Storage
- Storage Categories
- Tag
- Tax Value
- Web Mail Account
- Work Plan
- Work Plan Status
- Zone
Errors
The controllers actions will generate errors for the following cases:
- Status 400: Badly formed queries e.g. filter parameters that are not correctly encoded
- Status 401: Authentication failures e.g. unrecognised keys
- Status 403: Forbidden. The request was valid, but the server is refusing action. The user might not have the necessary permissions for a resource, or may need an account of some sort.
- Status 404: Not found. Unknown resources e.g. data which is not public
- Status 409: Conflict. Indicates that the request could not be processed because of conflict in the current state of the resource, such as an edit conflict between multiple simultaneous updates.
- Status 500: Server errors e.g. where something has gone
Errors are formatted in JSON
Versioning
It is possible to select the web services version using the {version} token
/api/{version}/{controller}/{details}/{action}/{id}?{querystring}
The token {version} can contain both "exact" values and the special "latest" alias, which identifies the most recent version among those existing in the system.
In general, the use of the special "latest" alias is strongly recommended.
If you want to be particularly "conservative" and adherent to a specific version, specify the name explicitly (eg "v1").
Routing
The system use the following routing syntax, consisting of a sequence of "path-tokens" (the request parameters):
{schema}://{host}/api/{version}/{controller}/{details}/{action}/{id}?{querystring}
The tokens identify respectively:
- {host} -> HOST of the URL
- {version} -> version of web services
- {controller} -> name of the service (controller) you want to invoke
- {details} -> optional detail level of the returned JSON (if applicable)
- {action} -> optional action (method) invoked in the controller
- {id} -> single optional argument (parameter) of the method in the controller, if it so requires
- {querystring} -> additional parameters and possible "modifiers" of the processing and serialization process
Options
The REST API functions implemented in CRM in Cloud include a vast set of options that allow you to adapt the structure and shape of JSON packages according to your needs and preferences.
Unlike the parameters, which are specified in the URL route (through tokens and queriestring), the options must instead be passed through the HTTP headers of the request.
As from RFC6648 all the options passed through HTTP headers have in their name the custom prefix Crm-.
If a certain option is not specified, the system will use the default value specific to the {version} indicated in the URL.
The available options are listed below, and the related accepted values are briefly described.
Global formatting options
The global formatting options allow a high level control on the behavior of the serializer/deserializer, determining the general characteristics of the returned/accepted JSON.
Crm-TypeNameHandling
- None: the JSON does not contain any type name for the objects represented
- Objects: the JSON contains the type names for the represented objects
- RootOnly: the JSON contains the type name of the represented object at root level
Crm-NullValueHandling
- Ignore: do not serialize the properties with NULL value
- Includes: also serialize the properties with NULL value
Crm-NamingStrategy
- AsIs: the properties are serialized with the same name as the source code
- UpperCamelCase: the naming convention "Pascal Case" is applied
- LowerCamelCase: the naming convention "Camel Case" is applied
- SnakeCase: the naming convention "Snake Case" is applied
- KebabCase: the naming convention "Kebab Case" is applied
Crm-Formatting
- None: the JSON is not indented, and results on a single line of text
- Indented: The JSON is indented (tabbing = 1)
Crm-WebApiRules is a comma-separated list of rules to activate. The currently supported values are:
- LeadMustBeUniqueByEmail=VALUE: When VALUE is true, the created Lead must be unique by email address. When VALUE is false (or the rule is not specified) the rule is ignored
Polymorphic Serialization
As already illustrated into the Options section, the REST API functions implemented in CRM in Cloud include a vast set of functions that allow you to adapt the structure and shape of JSON packages according to your needs and preferences.
See the Options section to get all the details related to their use
Polymorphic serialization options
The polymorphic serialization options act at a highly granular level, allowing a more "surgical" customization than is possible with the formatting options.
Click on an option in the table below to see how to use it (the "Crm-" prefix is omitted for the sake of brevity)
Note on enums: enum-typed properties are covered in a specific section
Polymorphic Enums
Many DTO properties are enum-typed.
In order to simplify their polymorphic serialization, a unified set of (optional) styles is available for each property:
- AdaptiveString: serialize the enum as string.
- AdaptiveInteger: serialize the enum as the equivalent numeric value
For more details about the polymorphic serialization of enums, please refer to the appropriate documentation
External References
Many Business Objects (and related DTO) of CRM in Cloud can be associated with an "external reference", ie the corresponding entity of another third party system.
The "external references" are therefore logical relationships between a native entity of CRM in Cloud and the corresponding entity of an external system.
They are to be seen as purely descriptive meta-data, and exist only for the benefit of the mappings made by the synchronization systems.
Typically "external references" are not involved in any internal CRM in Cloud process.
Example: companies and accounts
As an example, assume that CRM in Cloud is synchronized (via plugins or other mechanisms based on the REST API) with other N systems.
Each of these N systems will be discriminated by CRM in Cloud through an identifier specified in the Crm-ApplicationName parameter.
Each account (or any other Business Object) of CRM in Cloud may therefore have a "counterpart" for each of the N external systems.
The CompanyDTO.ownerId property represents the owner (an account of CRM in Cloud) of the company.
The following diagram illustrates the relationships described:
Polymorphic serialization of properties representing external references
The property CompanyDTO.ownerId can be serialized through various styles (see specific section on the polymorphic serialization of the "ownerId" field).
One of these serialization styles is AdaptiveExternalReference.
Using the style AdaptiveExternalReference, each "owner" (account) is represented by a string containing the username of the corresponding account in the calling client's database.
As previously mentioned, the correct value to return is determined using what is specified in Crm-ApplicationName.
GET
When making a GET request for a DTO, the property (supporting the AdaptiveExternalReference style) for which a polymorphic serialization option is specified, takes the following value:
- the string in the "External Reference Value" field (see previous diagram), if it is not null and not empty
- The name/code of the Business Object of CRM in Cloud in case "External Reference Value" (see previous diagram) is empty or null (or in the case no associations are present)
PUT / POST
When executing a PUT/POST request of a DTO, the property (supporting the AdaptiveExternalReference style) for which a polymorphic serialization option is specified, treats the received values as follows:
- If a string value is sent, it is used to resolve (in the "External Reference Value" field of the previous diagram) the relative integer value of the ID/PK of the "External Reference Table" record (associated with the table of the Business Object correspondent). If there is no record with the given string, an error is returned.
- If an integer is sent, it will be considered as the numeric ID previously mentioned (ID/PK of the "External References Table" of the previous diagram).
Emulation
As already illustrated into the Options section, the REST API functions implemented in CRM in Cloud include a vast set of functions that allow you to adapt the structure and shape of JSON packages according to your needs and preferences.
See the Options section to get all the details related to their use
Emulation options
If it is necessary to emulate the behavior of the previous version of the REST API (Tustena 17), it is possible (although not recommended) to use the following option.
- Crm-EmulateWebApiVersion
- Not specified: No emulation is activated
- v9: emulates the behavior of the previous generation REST API (Tustena 17)
OData
The REST APIs are internally based on the Microsoft WebAPI technology, and are largely compliant with the REST specifications, OData v3 and OData v4.
OData compliancy
There are several research methods based on OData.
The current implementation supports a significant portion of the OData v3 URL conventions and some OData v4 URL conventions and functions
OData criteria
In all OData-based search URLs, you can specify the following set of criteria:
- top
- skip
- orderby
- filter
- select
ID Search via OData
The following URL returns the IDs of the BOs found based on the specified criteria.
GET: /api/{version}/{controller}/SearchIds ?
- $filter = filter &
- $orderby = order criteria &
- $skip = items to skip &
- $top = max items to return
If invoked via POST, this URL allows you to specify the OData criteria by means of a JSON instead of using URL parameters (useful for avoiding data escaping).
Example:
"filter": "id eq 1 or (id gt 3 and id lt 10)",
"orderby": "id asc",
"top": "100",
"skip": "0",
"select": "id,companyName,billed"
Search for BO/DTO via OData
The following URL returns the DTOs in JSON format of the BOs found.
GET: /api/{version}/{controller}/Search ?
- $filter = filter &
- $orderby = order criteria &
- $skip = items to skip &
- $top = max items to return
If invoked via POST, this URL allows you to specify the OData criteria by means of a JSON instead of using URL parameters (useful for avoiding data escaping).
Example:
"filter": "id eq 1 or (id gt 3 and id lt 10)",
"orderby": "id asc",
"top": "100",
"skip": "0",
"select": "id,companyName,billed"
OData criteria diagnostics
The following URL allows you to test the OData parser, obtaining a JSON representing the OData parameter values passed in the querystring or POST.
GET: /api/{version}/{controller}/GetODataQueryParameters
$filter - logical operators
The expressions of the $filter parameter support the following logical operators:
- eq -> Equal
- ne -> Not Equal
- gt -> Greater Than
- ge -> Greater Than or Equal
- lt -> Less Than
- le -> Less Than or Equal
- and -> Logical And
- or -> Logical Or
For more details refer to the related OData v3 specifications
$filter - arithmetic operators
The expressions of the $filterparameter support the following arithmetic operators:
- add -> sum
- sub -> subtraction
- mul -> multiplication
- div -> division
- mod -> module
For more details refer to the related OData v3 specifications
Examples
- add -> /api/latest/Company/Search?$filter=Billed+add+5+eq+15
- sub -> /api/latest/Company/Search?$filter=Billed+sub+5+eq+25
- mul -> /api/latest/Company/Search?$filter=Billed+mul+5+eq+100
- div -> /api/latest/Company/Search?$filter=Billed+div+5+eq+5
- mod -> /api/latest/Company/Search?$filter=Billed+mod+5+eq+0
$filter - canonical functions
The expressions of the $filter parameter support the following canonical functions:
- startswith
- endswith
- length
- indexof
- replace
- substring
- substringof
- tolower
- toupper
- trim
- concat
For more details refer to the related OData v3 specifications
Examples
- startswith: /api/latest/Company/Search?$filter=startswith(companyName,'ACME')+eq+10
- endswith: /api/latest/Company/Search?$filter=endswith(companyName,'srl')+eq+10
- contains: /api/latest/Company/Search?$filter=contains(companyName,'srl')
- length: /api/latest/Company/Search?$filter=length(companyName)+eq+10
- indexof: /api/latest/Company/Search?$filter=indexof(companyName,'S')+eq+7
- replace: /api/latest/Company/Search?$filter= replace(companyName,'srl','s.r.l.')+eq+'acme+s.r.l.'&$top=10&$skip=0
- substring (1 argument): /api/latest/Company/Search?$filter=substring(companyName,1)+eq+'do'
- substring (2 arguments): /api/latest/Company/Search?$filter=substring(companyName,1,2)+eq+'do'
- substringof: /api/latest/Company/Search?$filter=substringof('srl',companyName)
- tolower: /api/latest/Company/Search?$filter=tolower(companyName)+eq+'my+company+name'
- toupper: /api/latest/Company/Search?$filter=toupper(companyName)+eq+'MY+COMPANY'
- trim: /api/latest/Company/Search?$filter=trim(companyName,1)+eq+'my+company'
- concat: /api/latest/Company/Search?$filter=concat(companyName,Code)+eq+'My+companyABC'
Related items
The WebApi controllers are able to detect OData parameters if they are specified in the URLs of requests for related items/children.
The current implementation supports:
- Top
- Skip
- OrderBy
- Filter
- Select
Here are some examples of applying OData to web services
Related items - top
Template URL -> GET: /api/{version}/{controller}/{id}/{SubItems}?$top=10
Esempio -> GET: /api/{version}/Company/1/Contacts?$top=10
Related items - skip
Template URL -> GET: /api/{version}/{controller}/{id}/{SubItems}?$skip=10
Esempio -> GET: /api/{version}/Company/1/Contacts?$skip=10
Related items - pagination
By combining $top and $skip it is possible to paginate the resultset
Template URL -> GET: /api/{version}/{controller}/{id}/{SubItems}?$top=10&$skip=50
Esempio -> GET: /api/{version}/Company/1/Contacts?$top=10&$skip=50
Related items - orderby
Template URL -> GET: /api/{version}/{controller}/{id}/{SubItems}?$orderby=criteria
Esempio -> GET: /api/{version}/Company/1/Contacts?$orderby=Id%20desc
Related items - filter
Template URL -> GET: /api/{version}/{controller}/{id}/{SubItems}?$filter=criteria
Esempio -> GET: /api/{version}/Company/1/Contacts?$filter=CompanyId%20eq%201
Related items - select
Template URL -> GET: /api/{version}/{controller}/{id}/{SubItems}?$select=csv
Esempio -> GET: /api/{version}/Company/1/Contacts?$select=id,companyName
Related items - other examples
GET:
/api/{version}/Company/1/Activities?$filter=Subject%20eq'Appuntamento'&$top=10&$skip=5&$orderby=Description%20desc
GET:
/api/{version}/Company/1/Contacts?$filter=Name%20eq%20'Damiano'
Primitive data types supported by OData
The OData parser supports the following primitive data types:
- integer numbers
- non integers
- strings
- bool
- date/time (see paragraph below)
Specifically, the supported MS.NET types are:
- System.Byte
- System.SByte
- System.UInt16
- System.Int16
- System.UInt32
- System.Int32
- System.Int64
- System.UInt64
- System.Single
- System.Double
- System.Decimal
- System.String
- System.DateTime (vedi paragrafo a seguire)
OData and the enum-typed properties
The OData parser supports several enum types, but remember that they must be expressed via the equivalent string value, not the native numeric value.
Examples:
GET:
/api/{version}/Company/1/Opportunities?$filter=status eq 'Suspended'
GET:
/api/{version}/Opportunity/SearchIds?$filter=status eq 'Suspended'
OData and the date/time properties
The OData parser supports date/time fields (System.DateTime).
It is possible to express a date/time in different ways (both in OData v3 and OData v4 format).
Here are some correct examples, and some incorrect examples.
Correct examples in OData v3 format:
- $filter=myDateTimeField eq datetime'2015-07-28T10:23:00Z'
- $filter=myDateTimeField eq datetime'2015-07-28T10:23:00.1Z'
- $filter=myDateTimeField gt datetime'2015-07-28T10:23:00.12Z'
- $filter=myDateTimeField lt datetime'2015-07-28T10:23:00.123Z'
- $filter=myDateTimeField eq datetime'2015-07-28T10:23:00.1234Z'
- $filter=myDateTimeField gt datetime'2015-07-28T10:23:00.12345Z'
- $filter=myDateTimeField eq datetime'2015-07-28T10:23:00.123456Z'
- $filter=myDateTimeField lt datetime'2015-07-28T10:23:00.1234567Z'
Correct examples in OData v4 format:
- $filter=myDateTimeField eq 2015-07-28T10:23:00Z
- $filter=myDateTimeField eq 2015-07-28T10:23:00.1Z
- $filter=myDateTimeField gt 2015-07-28T10:23:00.12Z
- $filter=myDateTimeField lt 2015-07-28T10:23:00.123Z
- $filter=myDateTimeField eq 2015-07-28T10:23:00.1234Z
- $filter=myDateTimeField gt 2015-07-28T10:23:00.12345Z
- $filter=myDateTimeField eq 2015-07-28T10:23:00.123456Z
- $filter=myDateTimeField lt 2015-07-28T10:23:00.1234567Z
Wrong examples:
- $filter=myDateTimeField eq '2015-07-28T10:23:00.1Z'
- $filter=myDateTimeField eq cast(2015-07-28T10:23:00Z)
Searchable and not searchable properties
Not all DTO properties are searchable via OData.
To check if a particular property is searchable, visit the specific documentation reference and check what is indicated under "Is Searchable".
Name | Value |
---|---|
... | |
Is Searchable | YES |
... |
Examples:
- Searchable property: CompanyDTO.address
- Non searchable property: CompanyDTO.boStatus
IMPORTANT NOTE
THE RESULT OF COMPARISONS BETWEEN STRINGS ARE DETERMINED BY THE COALESCE SETTINGS AT THE DATABASE LEVEL OF SQL SERVER.
IT FOLLOWS THAT, EVEN IF SOME FUNCTIONS OF ODATA ARE IMPLEMENTED, THEIR BEHAVIOR IS INFLUENCED BY SUCH SETTINGS AND MAY GENERATE UNINTENDED RESULTS.
IT IS NECESSARY THEREFORE TO CONSIDER THIS ASPECT WHEN EXECUTING OPERATIONS IN WHICH THE CASE-SENSITIVITY IS RELEVANT.
Free Fields
Many Business Objects (and related DTO) are customizable, and it is therefore possible to define for each a different set of custom fields.
These custom fields are called Free Fields.
Free Fields are user-definable custom fields, and can contain data of various kinds.
Each Free Field has a specific data type (selected during configuration), and on the basis of this type, the field data follow certain validation rules.
Free Field and polymorphism
As with many other fields, Free Fields also implement polymorphic serialization capabilities, and it is therefore possible to select multiple serialization/deserialization styles.
For more details, refer to the specific descriptive paragraph on the serialization of the Free Fields
Free Field and OData
Free Fields can be used in OData searches as field selectors ($select criterion).
In order to be able to discriminate them from the other standard fields of the Business Object, it is necessary to specify the prefix "FF_"
Example:
GET: /api/{version}/Company/Search?$select=id,companyName,FF_MyCustomFreeField1,FF_AnotherFreeField
Business Objects supporting Free Fields
The list below contains all the controllers related to Business Objects that support the definition and usage of Free Fields.
- Account
- Activity
- Address
- Catalog
- Catalog Product Price
- Company
- Contact
- Lead
- Opportunity
- Order
- Quote
- Storage
Glossary
Glossary of terms
Below is a list of the terms most used in these documents
Business Object -> The objects representing system entities (companies, contacts, leads, opportunities, activities, etc.) are called "Business Objects" (indicated for brevity with the acronym "BO"). For more details refer to the introductory section
BO -> Acronym used to refer to "Business Objects". For more details refer to the introductory section
DTO -> Acronym of Data Transfer Objects. For each Business Object there is a corresponding DTO. The purpose of DTO is to represent, serialize and transport the data of a Business Object. For more details refer to the introductory section
Controller -> Portion of the URL of routing of an end-point API REST. See also "routing". For more details refer to the introductory section
Action -> RPC functionality of a controller, invocable via the routing URL of an endpoint API REST. See also "routing". For more details refer to the introductory section
Polymorphic Serialization -> The polymorphic serialization options act at a highly granular level, allowing a more "surgical" customization than is possible with the formatting options. Some properties of DTOs are marked as "polymorphic". For these properties it is possible to specify a serialization style, which changes its JSON form and structure. For more details, refer to the options section
Logical Primitive -> Some properties of DTOs are marked as "Logical Primitive". The following data types are considered "Logical Primitive":
- Strings
- Numbers (both integers and decimals)
- Dates (and time intervals)
- Boolean
- Enums (both in integer an string format)
Business Object Type -> the complete list of Business Objects is formalized in the appropriate enum "BusinessObjectType". For more details refer to the introductory section
Foreign Key -> Foreign key, or "foreign key". Some properties of DTOs are marked as "Foreign key". These properties are used to contain the association value (typically a numeric ID) between an object/DTO/BO "master" and a related object "detail".
List -> Some properties of DTOs are marked as "Foreign key". These properties are used to contain the association value (typically a numeric ID) between an object/DTO/BO "master" and a related object "detail". Some "Foreign keys" may contain a link to a list of objects/DTO/BO
Virtual Property / Aliased Property -> An alias for a physical property of a DTO/BO. I.e., the property "NormalizedEmail" of CompanyDTO is serialized as "emails"